Binary
Binary
Lesson Objectives
- The binary representation of numbers
- Understand the units of computer memory
- Converting denary numbers to a binary system and vice versa
- Adding binary numbers
- Binary shifts
- Bitwise operations
- Representing negative numbers in a binary system
- Applications of the binary system
Introduction
- A computer has many electronic components that work as switches.
- These components have two logics as input and output: ON and OFF.
- A similar logic is used to represent data in binary form. ON is represented as 1, and OFF is represented as 0.
Place value of the denary system
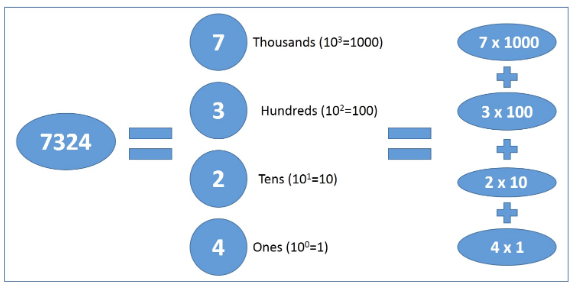
- The denary system has a base value of 10.
- It counts in multiples of 10.
Place value of the binary system
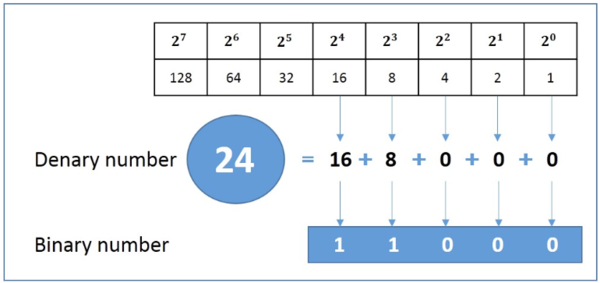
- The place values of binary numbers are of base 2.
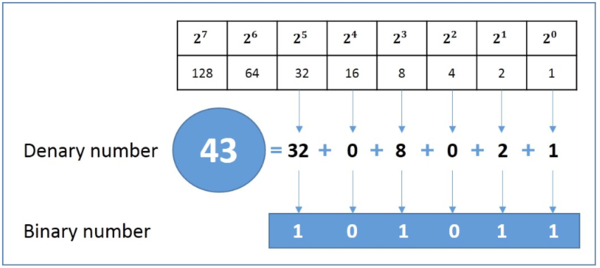
Size of computer memory: base-2
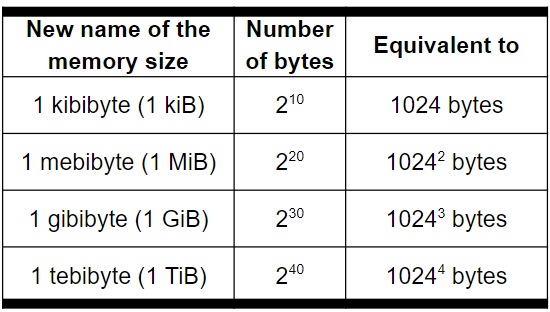
- A binary digit is referred to as a bit.
- A nibble consists of 4 bits.
- A byte consists of 8 bits.
- A byte is the smallest unit of memory in the computer system.
- The memory sizes available with computers are in multiples of 8 such as 16-bit systems, 32-bit systems, etc.
- The memory sizes were originally standardised using the base-2 representation.
- In this system, the prefixes kibi-, mebi-, gibi-, tebi- are used to avoid conflicts with the base-10 system.
- This representation is now used for representing the size of RAM modules only.
Size of computer memory: base-10
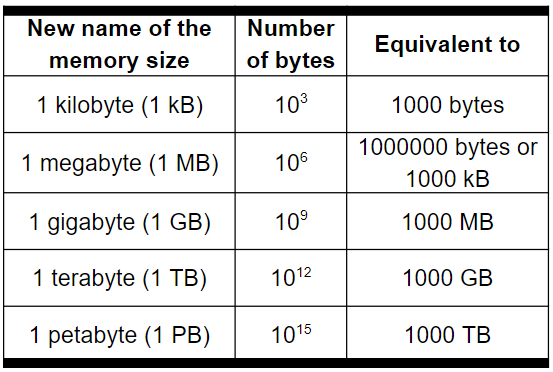
- After the standardization of base-10 representation, the memory sizes are now represented as given.
Converting denary to binary
- A bidirectional bus consisting of 8, 16, 32, and 64 parallel lines.
- This bus is used to transmit data and instructions between the processor, memory, and input-output devices.

- A denary number is converted to binary by dividing it by 2 and calculating the remainders.
- The binary equivalent is obtained by arranging the remainders in reverse order.
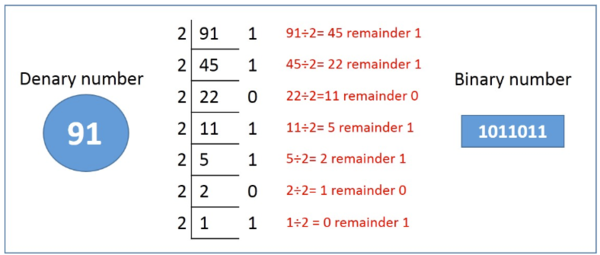
How do you check your answer?
- Binary equivalent of denary number 91:
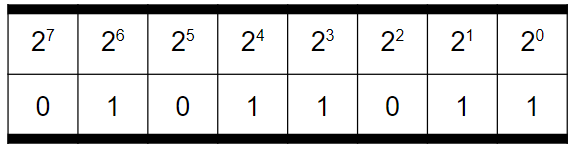
- The answer can be checked by:
(0×128)+(1×64)+(0×32)+(1×16)+(1×8)+(0×4)+(1×2)+(1×1)=91
Binary combinations
- A one-bit system has a one-place value and can have 2 possible combinations: 0 or 1.
- Similarly, a 2-bit system has two-place values and has 4 possible combinations, as shown in the table.
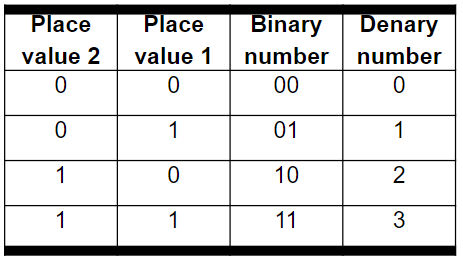
- Similarly, a 3-bit system has three-place values and has 8 possible combinations.
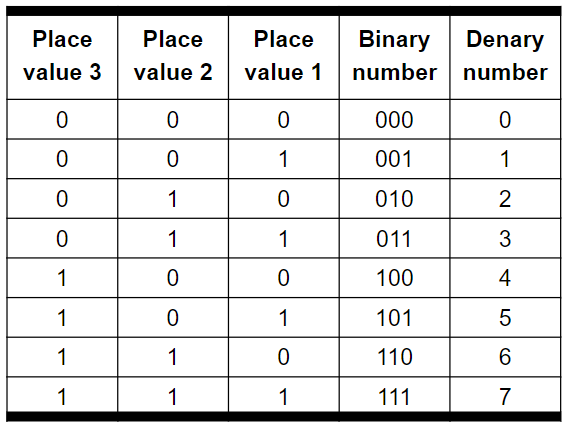
Representing numbers
- Programmers use many arithmetic operations in a program.
- The numbers are either represented as integers or floating point numbers.
- Integers are whole numbers, and floating point numbers are used to represent numbers with decimal points.
- A 16-bit system can represent integers up to 216-1=65535.
- 8-bit, 16-bit, 32-bit, and 64-bit are the most common bit lengths.
Adding binary numbers
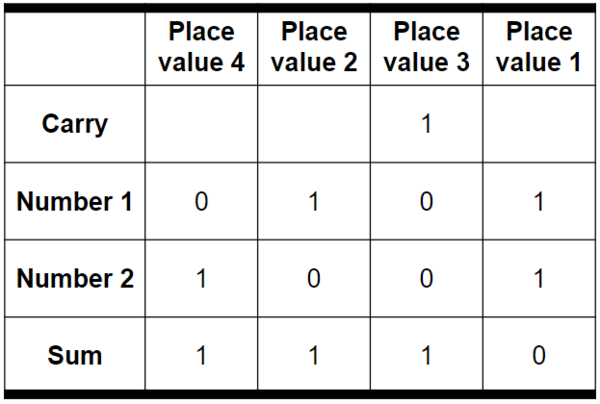
- Binary numbers are added in the column method as the denary numbers are added.
- Adding 0101 and 1011 in the table.
- Adding binary numbers:
0+0=0
1+0=1
1+1=10 (1 is carried over)
How to check your answer
- 0101 and 1001 represent the denary numbers 5 and 9.
- The sum of 5 and 9 is 14.
- Convert the sum obtained to a denary number.
- (8×1)+(4×1)+(2×1)+(0×1)=14
The rules are:
- 0+0=0
- 1+0=1 (sum 1 and carry 0)
- 1+1=10 (sum 0 and carry 1 )
- 1 + 1 + (carry 1) = 11= sum 1 and carry 1
Adding three binary numbers
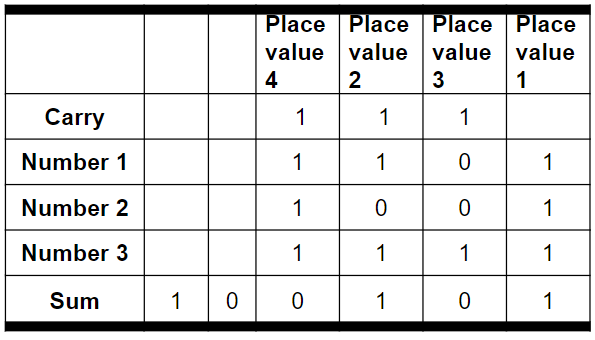
The rules are:
- 1 + 0 + 0 = sum 1 and carry 0
- 1 + 1 + 0 = sum 0 and carry 1
- 1 + 1 + 1 = sum 1 and carry 1
- 1 + 1 + 1 + (Carry 1) = 1 0 0
- Adding four 1’s results in a sum of 0, and carry 1 is placed two places to the left.
Overflow error
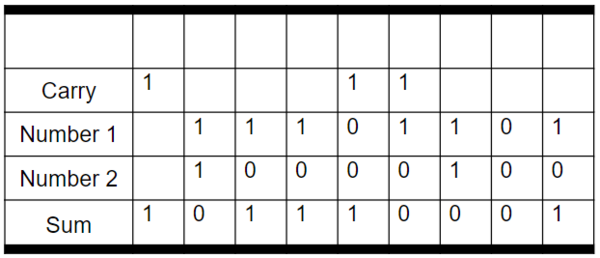
- A CPU with an 8-bit register has a capacity of up to 11111111 in binary. If an extra bit is added, it is said to be an overflow error.
- The number of bits a register can hold is called word size. Exceeding the capacity of word size in a register results in an overflow error.
- Consider the addition of two binary numbers 11101101 and 10000100.
- The sum of these two numbers is bigger than 8 bits (an extra bit than the register can hold). The computer thinks that 11101101+10000100=01110001 as it does not have space to store the extra bit.
Binary Shifts
- Let us consider the denary number 6. Its binary equivalent is 0110.

- What is the binary equivalent of 12? Is it in any way related to the binary equivalent of 6?
- The binary equivalent of 12 (6 × 2) is:

- It can be noted that the binary equivalent of 6 shifted to the left by one place is the binary equivalent of 12.
- The binary equivalent of 12 is:

- The binary equivalent of 24 is:

- Again, it can be noted that the binary equivalent of 12 shifted to the left is the binary equivalent of 24.
- It can be summarised that when a denary number is multiplied by 2, its binary equivalent shifts left by 1 place.
- Also, multiplication by 4 results in a shift of binary equivalent by 2 places.
- Multiplication by 8 results in a shift of binary equivalent by 3 places and so on.
Representing negative numbers
- Signed integers can be either positive or negative.
- An extra bit is used to represent the sign of a number in binary representation.
- In the case of signed numbers, the leftmost bit is used to represent the sign and is called the sign bit.
- 0 represents a positive number, and 1 represents a negative number.
- The rest of the bits represent the magnitude of the number.
- Consider the 8-bit binary number 10001101.
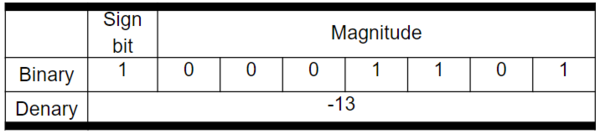
- The smallest number that can be represented using 8 bits is 11111111 (-127), and the largest number is 01111111 (+127).
- Similarly, the signed number can be represented in 32-bits, 64-bits, and so on.
Finding two's complement
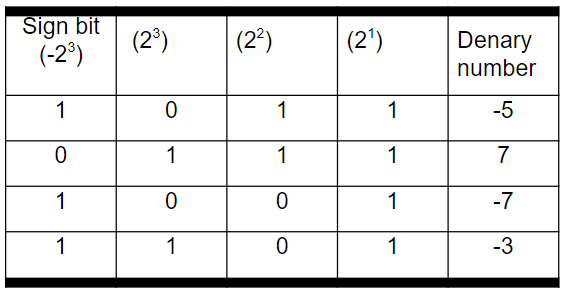
- Finding two’s complement is an alternate method to represent negative numbers. This method is used by most computers to perform mathematical operations.
- Let us consider an example of representing -5. The binary value of 5 is 101. The leftmost bit is added to represent the positive sign. +5 is 0101.
- Each bit is inverted and, hence, the 0101 becomes 1010. 1 is added to this number. 1010 + 1=1011.
- Some examples are given.
Sum of numbers: Using two’s complement
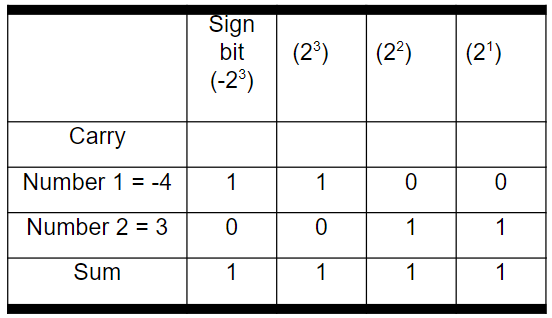
- Let us consider adding -4 and 3 using two’s complement.
- Converting the sum 1111 into a denary number,
-8+4+2+1=-1
Applications of the binary system
- Microprocessors in computer use registers to control devices such as robots.
- Registers store a group of bits.
- Consider a robotic vehicle that has four wheels.
- Register used to control this robotic vehicle:
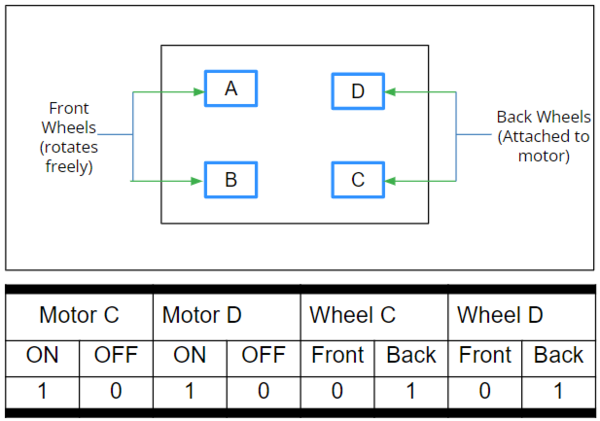
- For example: A value of 10100101 means ‘ Motor C is ON, and Motor D is ON, and both wheels are moving together backward.’
- As a result of these values, the robotic vehicle moves backward.
Related Worksheets: